Fields
Fields can be added from the Entity Options panel (you can open this panel by clicking on the Entity). Vemto supports the majority of Laravel Migrations field types and indexes.
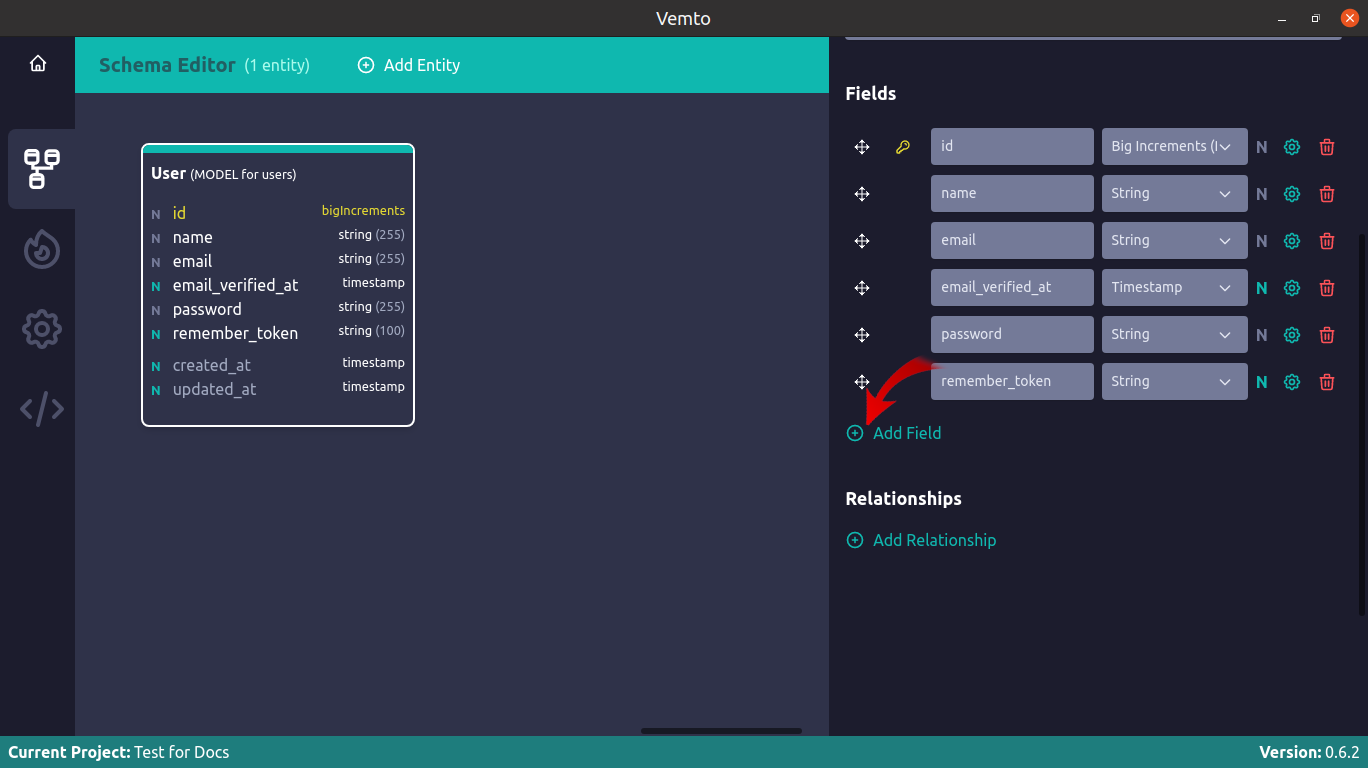
Creating
To add a new field, you can click on the Add Field button under the Entity Options. Then, just type the name and choose the type.
{warning} It is highly recommended to use lower case field names
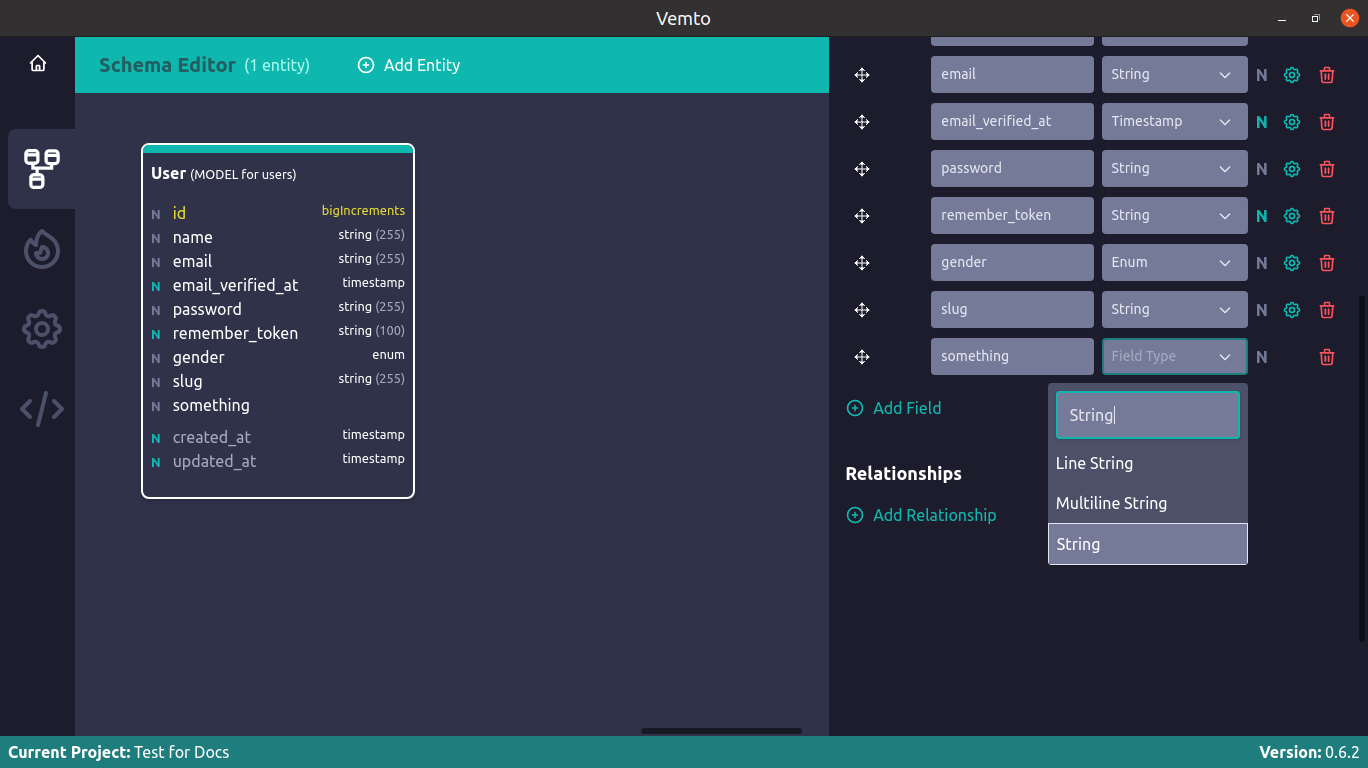
{info} Vemto has a huge internal Fields Library and will try to automatically identify the field type, the faker, and the options based on the field name you wrote. For example, if you type "slug" on the field name, and the field doesn't have a type yet, Vemto automatically will suggest the String type, and will add the "$faker->slug" to the Faker property on the field options.
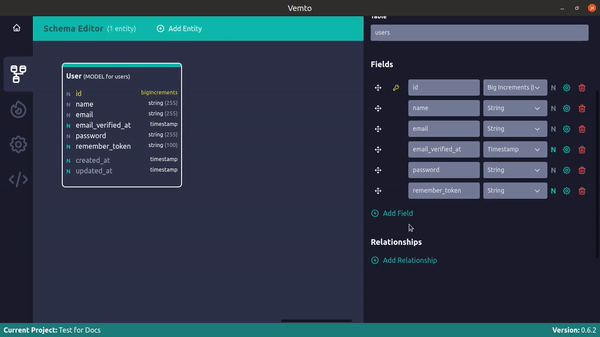
An example of Vemto automatically suggesting the field type and options
Field Options
The field options are accessible by clicking on the gears icon right after the field type.
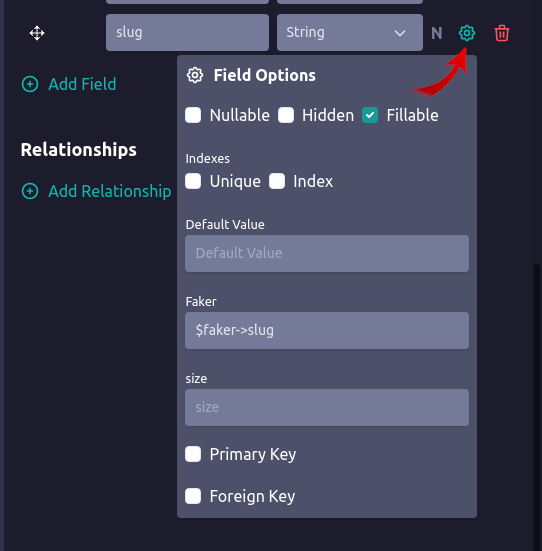
The available options are:
- Nullable - Defines if the field is nullable or not
- Hidden - Defines if the field is hidden or not (for example, the password field is hidden by default)
- Fillable - Defines if the field is fillable or not (this option is visible when the project uses the Fillable Mass Assignment strategy)
- Guarded - Defines if the field is guarded or not (this option is visible when the project uses the Guarded Mass Assignment strategy)
{info} If you change the Mass Assignment strategy from "Fillable" to "Guarded", Vemto automatically takes care of it and all the fields mass assignment will be updated accordingly
- Unique - Defines if the field has a unique index
- Index - Defines if the field has a plane index
- Default Value - Sets the field default value
- Faker - Defines the field faker to seed dummy data and run the tests
- Size - Defines the field size
- Options - Defines the field sizes (only for Enum and Set field types)
- Primary Key - Defines if the field is a primary key
{danger} Entities can have only one Primary Key. If you change a field to a primary key type (ID, Big Increments, etc) or mark it as Primary Key, Vemto will remove the primary key constraint from the latest PK and will update all relationships and foreigns that relates to it to point to the new PK
- Foreign Key - Defines if the field is a Foreign Key
Special faker definition
Besides supporting faker, the fields support some special faker definitions too:
- PHP Code - you can set a faker as a custom PHP code. For example, a code that randomly set a value for each time you run your seeders or factories:
array_rand(array_flip(['apple', 'banana']), 1)
- '{DEFAULT_OR_FIRST}' - use this value on your faker to seed with the field default value, or optionally the first option if you are dealing with an ENUM field (it will use the first option if the field has no default value)
- {SIZE} - use this option to replace some section of your faker with the field size. For example, if you have a string field with a size of 64 characters, you can use Str::random({SIZE}) on your faker, which will be translated to Str::random(64) on the generated code
- Fixed Value - you can use a fixed value too. Just add it to the faker input on your field options. Please remember to add quotes (' or ") if you are dealing with string values.
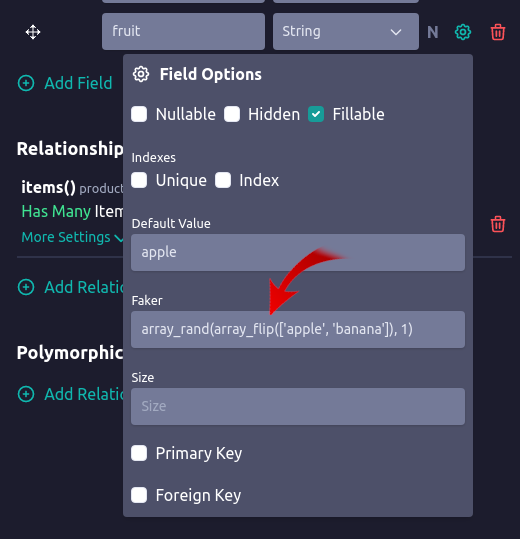
Foreign Key
When marking a field as Foreign Key, the following options are available:
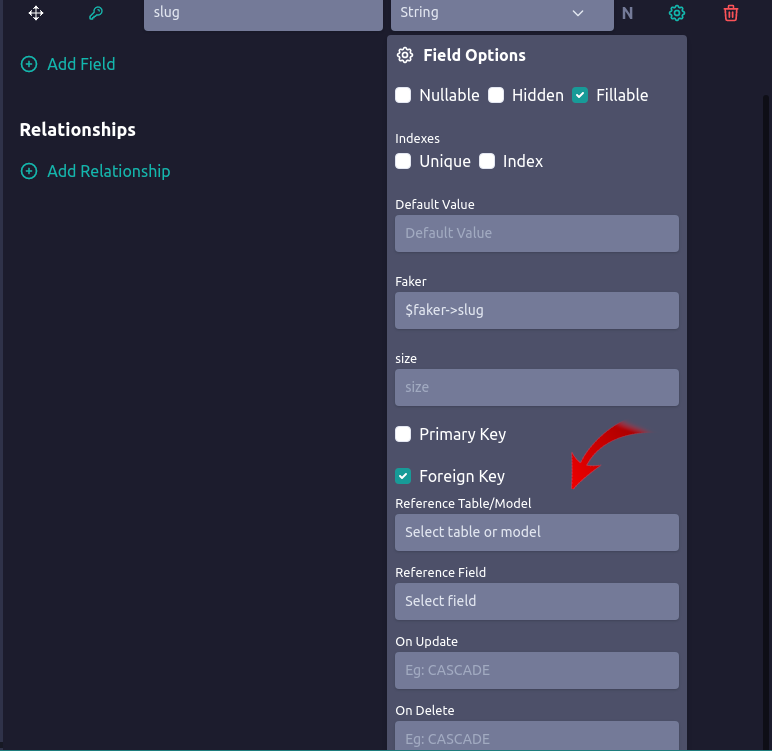
- Reference Table/Model - Defines the foreign referenced table or model
- Reference Field - Defines the foreign referenced field
- On Update - Defines the SQL action to run when updating the foreign data. E.g. CASCADE
- On Delete - Defines the SQL action to run when deleting the foreign data. E.g. CASCADE
{warning} It is highly recommended to follow the Eloquent Model Conventions when creating foreign keys