How to create a Laravel Rest API in minutes with Vemto
With Vemto, you can create a fully functional Laravel REST API, with authentication (provided by Laravel Sanctum), tests, and even nested resource endpoints, with just some steps.
This post assumes you have a basic knowledge of Laravel.
If you don't have Vemto installed on your machine, you can download a trial version here.
Let's start creating a project with this simple schema:
As you can see, we have three models and one table with some relationships:
- User - a user can have many pets and belongs to many categories (through the category_user table)
- Pet - A pet belongs to a user (represented by the user_id foreign key)
- Category - A category belongs to many users(through the category_user table)
In the GIF below you can watch the whole process of creating the schema above using the Vemto Schema Editor (the User model is automatically added when creating a new project):
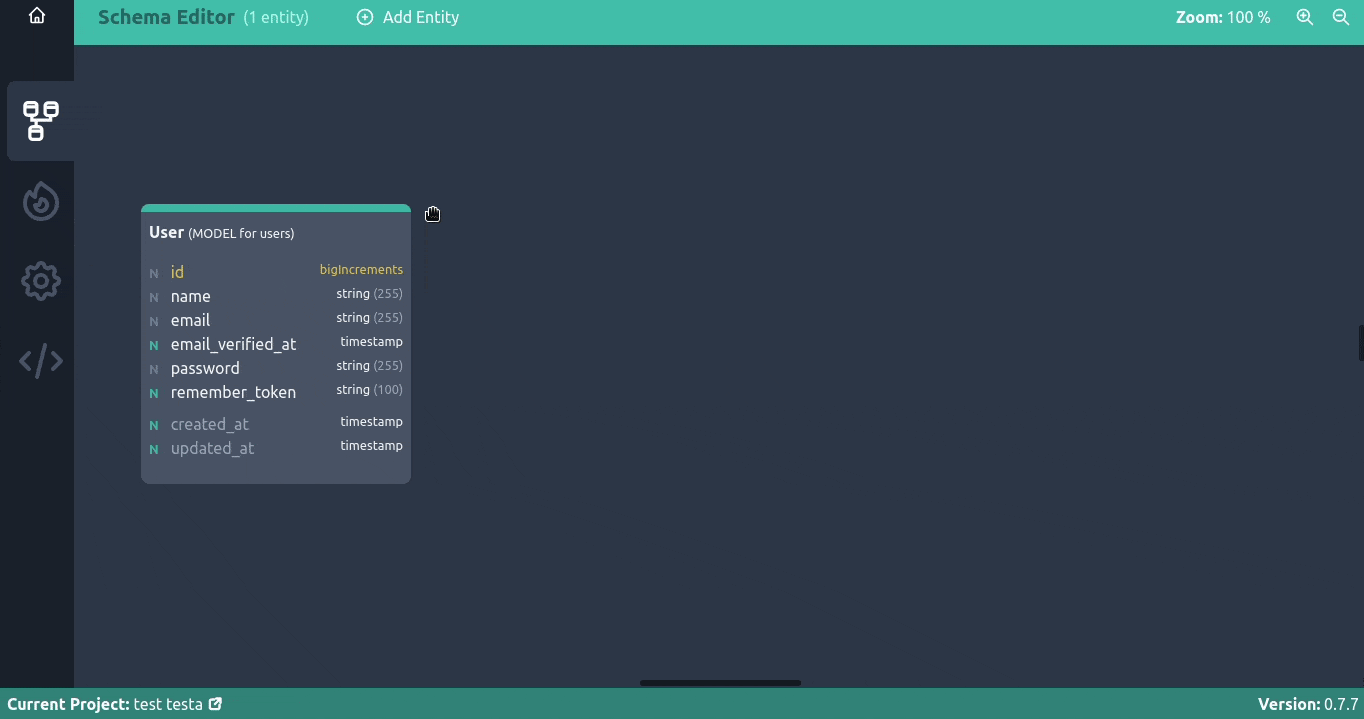
Adding Applications
Now that we have the schema definition, let's create some CRUD applications to represent our models.
You can do it by accessing the Applications Tab:
In our case, we'll add an application for each model we have created:
Accessing these applications, you can see an API tab where you can select what RESTful endpoints do you want to generate for this CRUD resource:
That is basically all you need.
Now, let's open the Generate Code tab and start the code generation (you can select the Only API option if you don't want to generate a full application with GUI):
Running the API
Our API is now ready.
Vemto takes care of generating all the necessary files (migrations, models, seeders, resources, controllers, tests, etc).
The authentication uses Laravel Sanctum, and you can get a token sending the email and password to the /api/login endpoint.
You can hit the Run button to run the migrations and start a small PHP server to test it (generally it will start on the https://localhost:8000 address). Or you can do it manually if you prefer.
In the GIF below you can see the API working using the Postman Client:
And here is an example of a controller file generated for our API:
<?php
namespace App\Http\Controllers\Api;
use App\Models\User;
use Illuminate\Http\Request;
use App\Http\Resources\PetResource;
use App\Http\Controllers\Controller;
use App\Http\Resources\PetCollection;
class UserPetsController extends Controller
{
/**
* @param \Illuminate\Http\Request $request
* @param \App\Models\User $user
* @return \Illuminate\Http\Response
*/
public function index(Request $request, User $user)
{
$this->authorize('view', $user);
$search = $request->get('search', '');
$pets = $user
->pets()
->search($search)
->latest()
->paginate();
return new PetCollection($pets);
}
/**
* @param \Illuminate\Http\Request $request
* @param \App\Models\User $user
* @return \Illuminate\Http\Response
*/
public function store(Request $request, User $user)
{
$this->authorize('create', Pet::class);
$validated = $request->validate([
'name' => ['required', 'max:255', 'string'],
]);
$pet = $user->pets()->create($validated);
return new PetResource($pet);
}
}
Conclusion
That's it.
Vemto is a powerful application for Laravel developers. It allows you to prototype a Restful API in minutes, saving your precious time to work on tasks that really need your care and effort.
Cheers